Immediately Invoked Function Expression (IIFE)
Published Date June 11, 2022
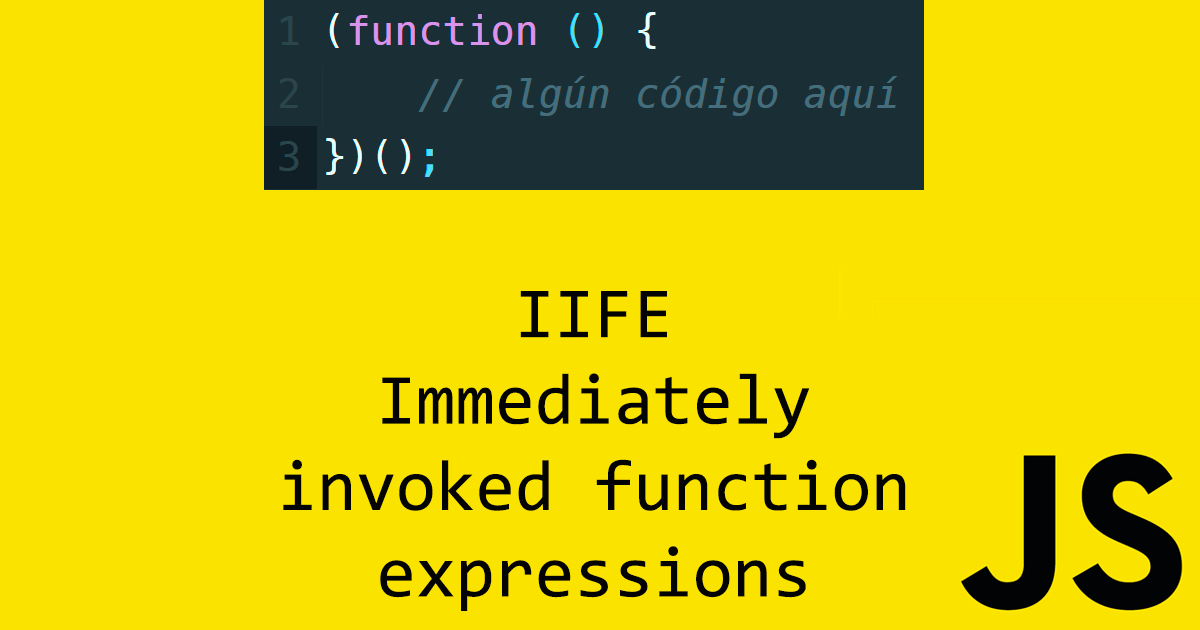
An immediately invoked function expression or IIFE, is a function written in javascript that is… invoke immediately as soon as it is defined.
IIFE stands for Immediately Invoked Function Expression, and it is a common programming pattern in JavaScript. It involves wrapping a function in parentheses, which then creates a function expression. The function expression is then immediately invoked using the "()" operator.
Here is an example of an IIFE:
(function() {
// code here
})();
In this example, the function is immediately invoked and the code inside the function is executed. This is a useful technique for initializing variables and executing code once when a script is loaded.
IIFEs can also take arguments and return values, just like regular functions. For example:
var result = (function(num1, num2) {
return num1 + num2;
})(3, 4);
console.log(result); // output: 7
In this example, the IIFE takes two arguments and returns their sum. The function is immediately invoked and the result is assigned to the "result" variable.
Overall, IIFEs are a useful tool for creating self-contained, modular code in JavaScript. They allow for better variable scoping and encapsulation, and can help improve the organization and readability of your code.